Due to user needs, I have to import one project to another one as a sub-project. The final result should be like the following picture.
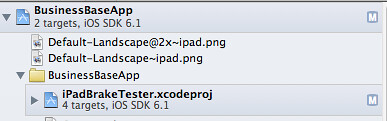
It is not easy to do this. We need some steps.
- Step 1, Add “iPadBrakeTester.xcodeproj” file to BusinessBaseApp project, note that don’t choose the “Copy items into…” checkbox.
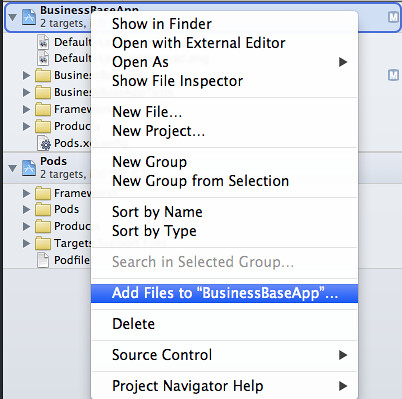
Step 2, close the iPadBrakeTester project, because you have opened the project in BusinessBaseApp project. If you don’t, you can just see the “iPadBrakeTester.xcodeproj” file and there is no triangle front of it, you will can’t expand it.
Step 3, generate static library. Choose “iPadBrakeTester.xcodeproj”,add Target. After generating static library, add .m, .h and .framework files to it.
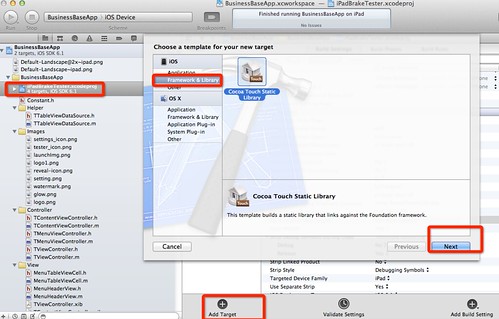
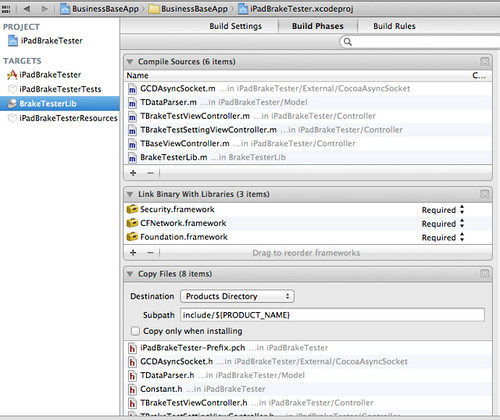
- Step 4, generate Bundle, and change the relevant settings to iOS target.
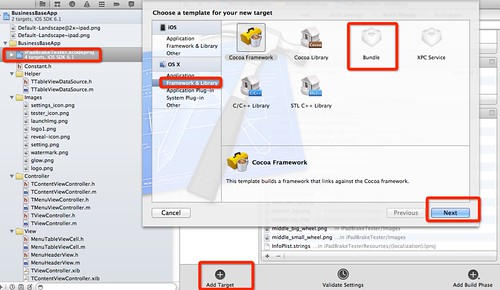
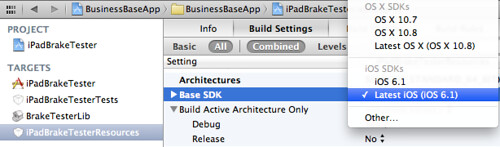
Then, add .xib and iamge files to it, like this:
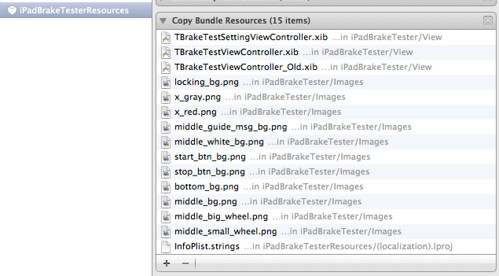
- Step 5, Use static library and Bundle in BusinessBaseApp project.
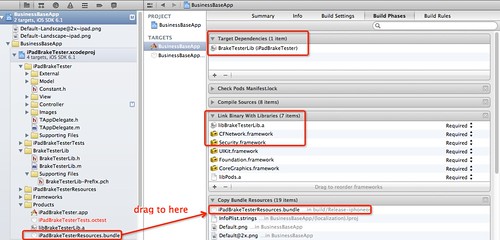
“Target Dependencies” means that it needs to rebuild the app object code if code in the dependency changes.
In “Link Binary With Libraries”,beside adding the static library, you need add the .framework files which you import to the sub-project.
Step 6, add “-ObjC” and “-all_load” flags to “Other Linker Flags”.
-ObjC because otherwise the linker does not properly load your classes.
-all_load if you have categories in the library, because otherwise those are not loaded.
Step 7, add the sub-project path to “User Header Search Paths”, like this:
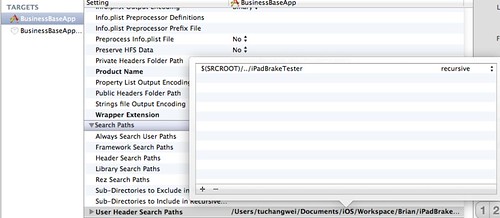
- Step 8, use Bundle in code.
1 |
|
Some references: